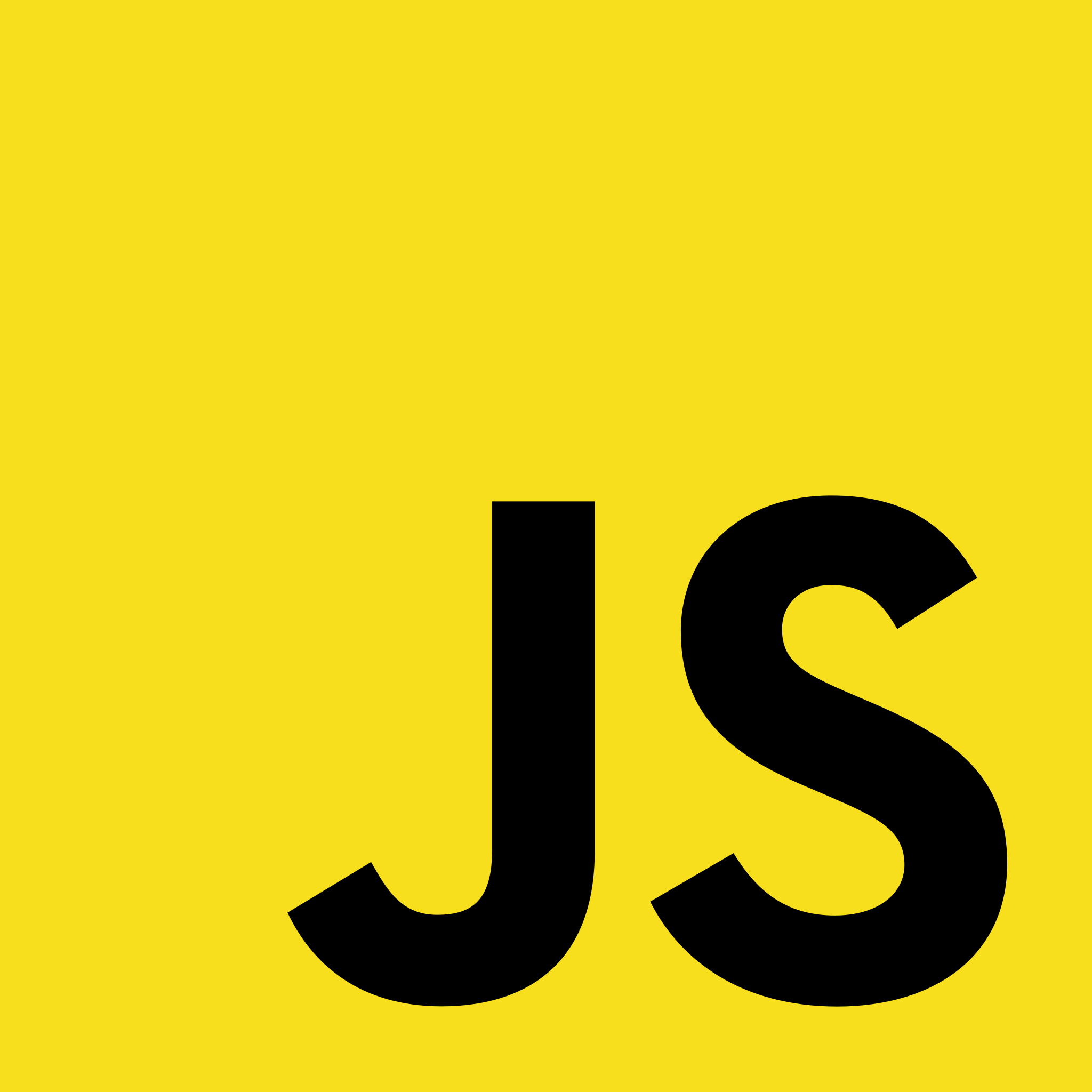
Algorithms and Data Structures – Chunk
--- Directions
Given an array and chunk size, divide the array into many subarrays
where each subarray is of length size
--- Examples
chunk([1, 2, 3, 4], 2) --> [[ 1, 2], [3, 4]]
chunk([1, 2, 3, 4, 5], 2) --> [[ 1, 2], [3, 4], [5]]
chunk([1, 2, 3, 4, 5, 6, 7, 8], 3) --> [[ 1, 2, 3], [4, 5, 6], [7, 8]]
chunk([1, 2, 3, 4, 5], 4) --> [[ 1, 2, 3, 4], [5]]
chunk([1, 2, 3, 4, 5], 10) --> [[ 1, 2, 3, 4, 5]]
Solution 1.
- Create to empty array to hold chunk called chunked
- For each element in the "chunked"
2-1. Retrieve the last element in "chunked"
2-2a.if last element does not exist, or its length is equal to the chunk size
push a new chunk into "chunked"with the current element
2-2b.else add the current element into the chunk
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | function chunk(array, size) { const chunked = []; for (let element of array) { const last = chunked[chunked.length - 1]; if (!last || last.length === size) { chunked.push([element]); } else { last.push(element); } } return chunked; } | cs |
Solution 2.
- Create empty "chunked"array
- Create index start at 0
- While index is less than array.length
3-1. Push a slice of length 'size' from 'array ' into 'chunked'
3-2. Add 'size' to 'index'
1 2 3 4 5 6 7 8 9 10 11 12 | function chunk(array, size) { const chunked = []; let index = 0; while (index < array.length) { chunked.push(array.slice(index, index + size)); index += size; } return chunked; } | cs |