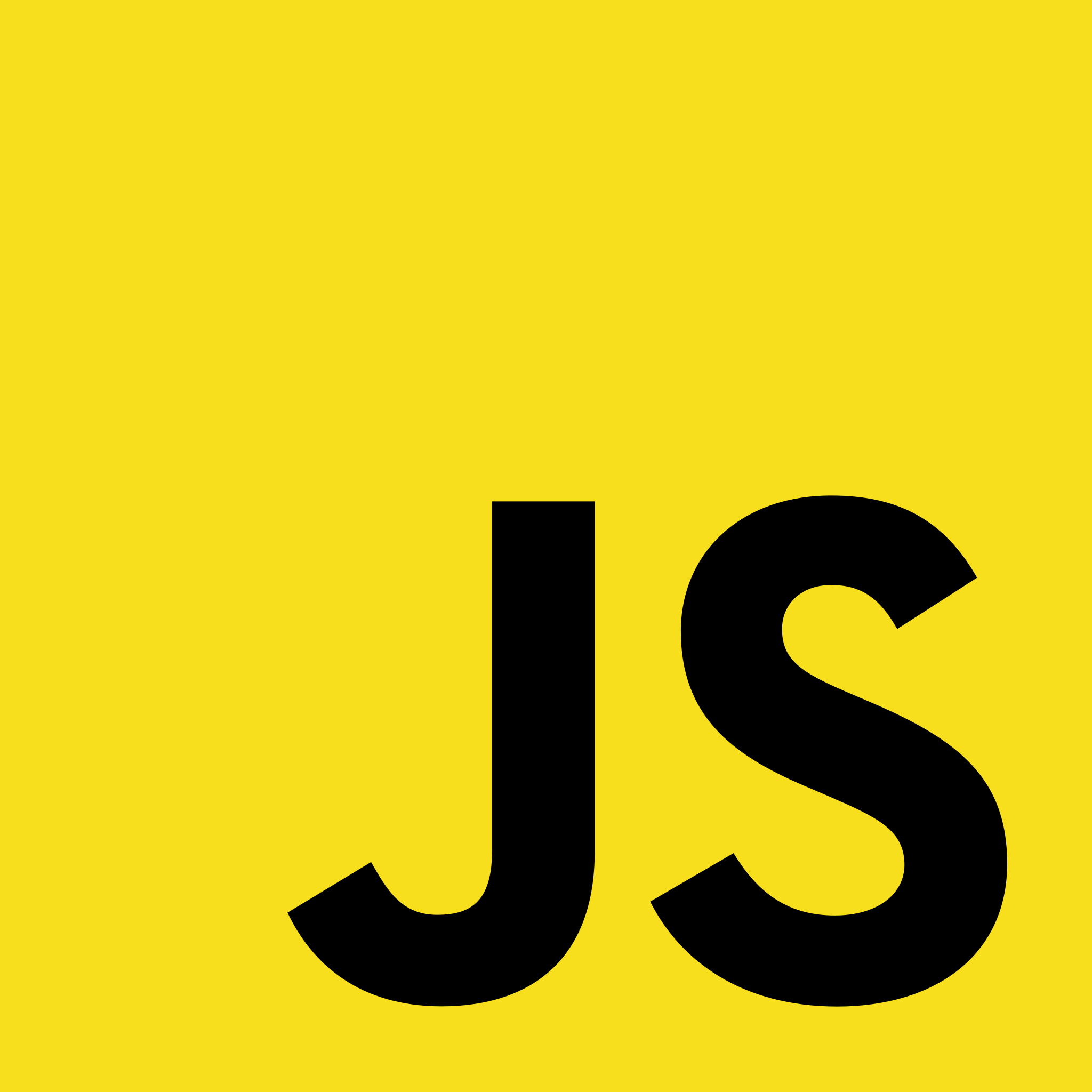
Algorithms and Data Structures – Capitalization
--- Directions
Write a function that accepts a string. The function should
capitalize the first letter of each word in the string then
return the capitalized string.
--- Examples
capitalize('a short sentence') -->'A Short Sentence'
capitalize('a lazy fox') -->'A Lazy Fox'
capitalize('look, it is working!') -->'Look, It Is Working!'
Solution 1.
- Make a empty array 'words'
- Split the input string by space to get an array
- For each word in the array
3-1. Uppercase the first letter of the word
3-2. Joint first letter with rest of the string
3-3. Push result into 'words' array - Join 'word' into string and return it
1 2 3 4 5 6 7 8 9 | function capitalize(str) { const words = []; for (let word of str.split(' ')) { words.push(word[0].toUpperCase() + word.slice(1)); } return words.join(' '); } | cs |
Solution 2.
- Create empty string called 'result'
- for each character in the string
2-1. IF the character to the left a space
Capitalize it and add it to 'result'
2-3. ELSE
add it to 'result
1 2 3 4 5 6 7 8 9 10 11 12 13 | function capitalize(str) { let result = str[0].toUpperCase(); for (let i = 1; i < str.length; i++) { if (str[i - 1] === ' ') { result += str[i].toUpperCase(); } else { result += str[i]; } } return result; } | cs |