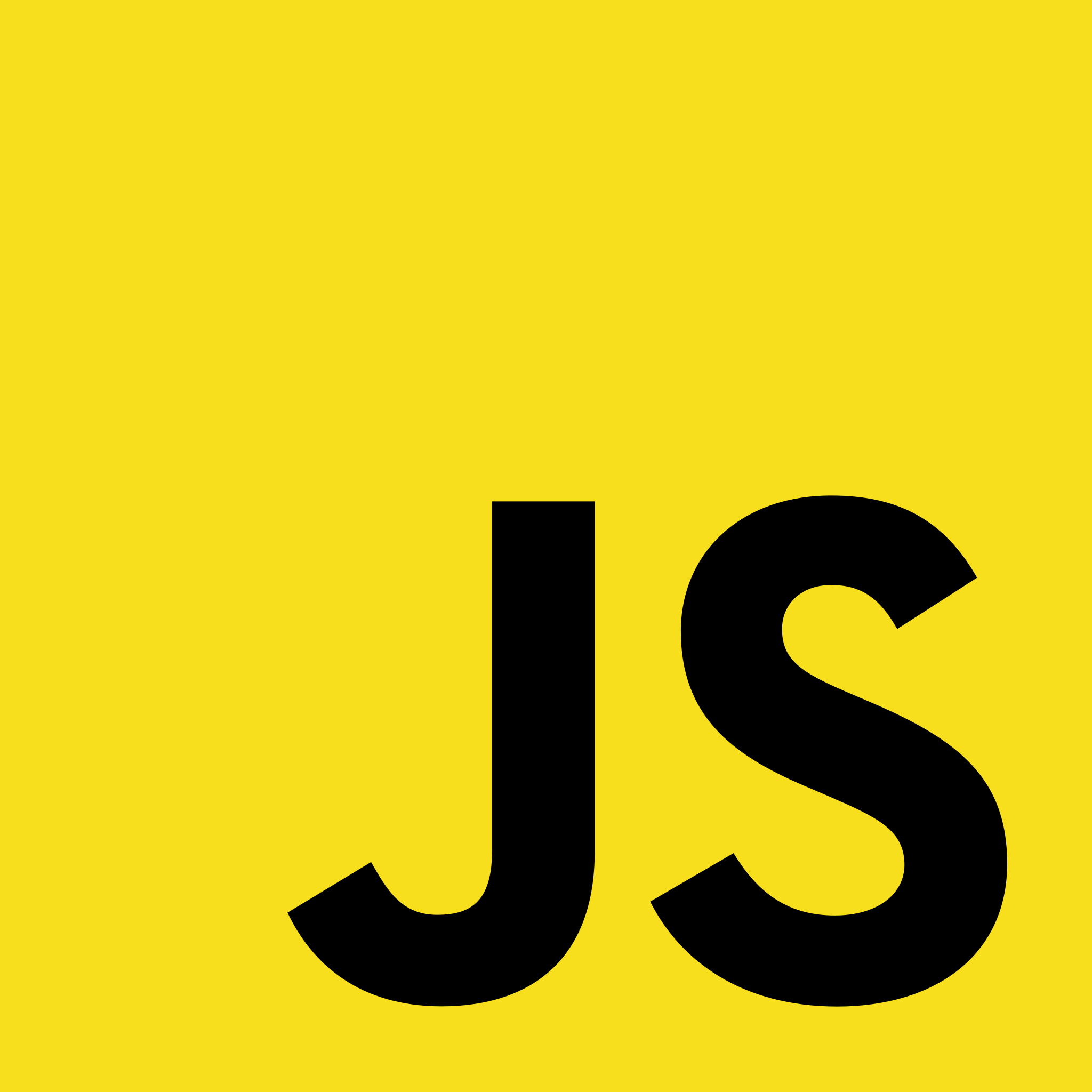
Algorithms and Data Structures – Palindrome
--- Directions
Given a string, return true if the string is a palindrome
or false if it is not. Palindromes are strings that
form the same word if it is reversed. *Do* include spaces
and punctuation in determining if the string is a palindrome.
--- Examples:
palindrome("abba") === true
palindrome("abcdefg") === false
Solution 1
1 2 3 4 5 6 | function palindrome(str) { const reversed = str.split('').reverse().join('') return str === reversed; } | cs |
Solution 2
- Using an array
- compare each items
[0, 10, 15] -> is every value greater than 5?
array.every((val)=> val > 5);
1 2 3 4 5 | function palindrome(str) { str.split('').every((char, i) => { return char === str[str.length - i - 1] }) } | cs |