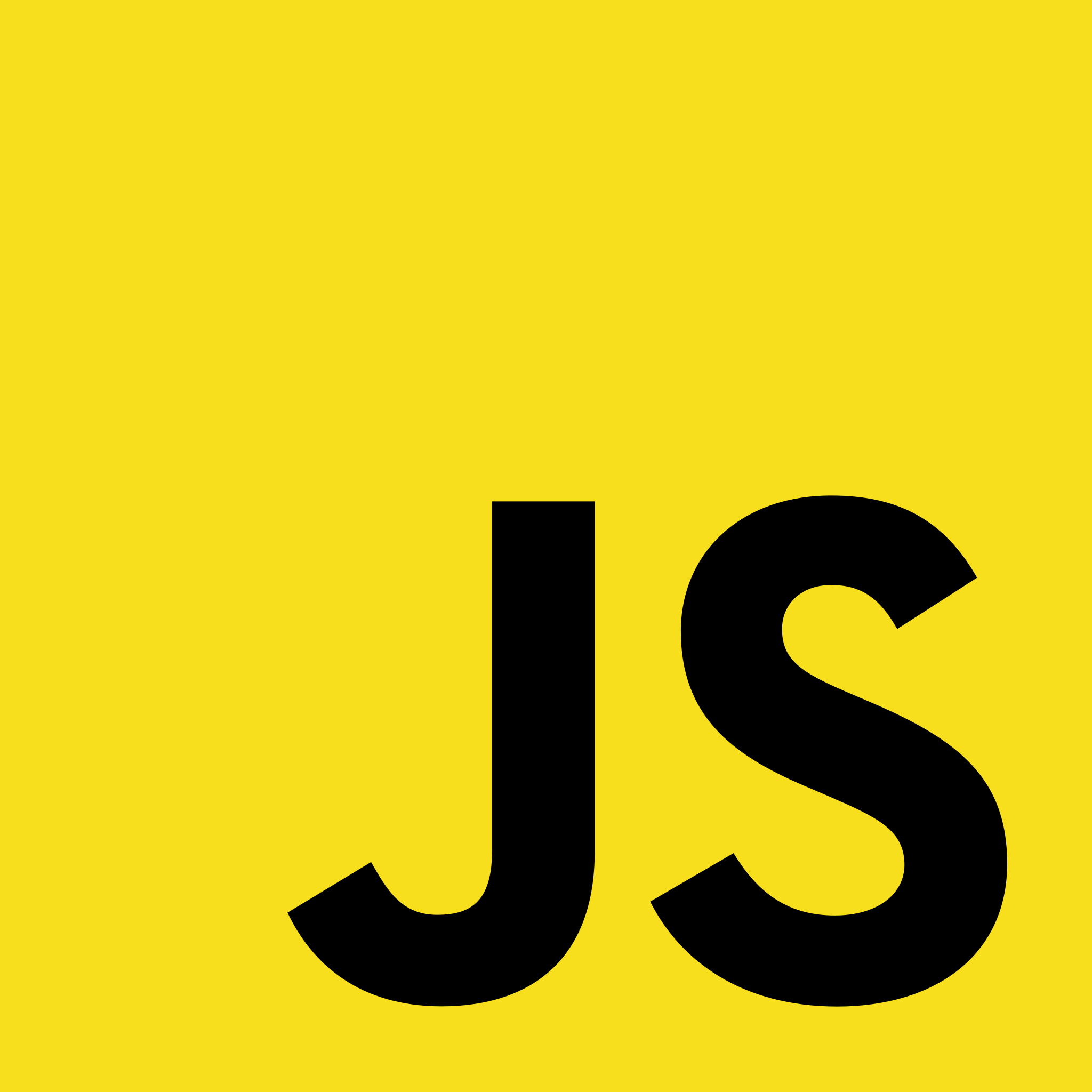
Algorithms and Data Structures – Spiral Matrix
--- Directions
Write a function that accepts an integer N
and returns a NxN spiral matrix.
--- Examples
matrix(2)
[[1, 2],
[4, 3]]
matrix(3)
[[1, 2, 3],
[8, 9, 4],
[7, 6, 5]]
matrix(4)
[[1, 2, 3, 4],
[12, 13, 14, 5],
[11, 16, 15, 6],
[10, 9, 8, 7]]
1. How to Approach
- Create empty array of arrays called 'result'
- Create a counter variable, starting at 1
- As long as (start column <= end column) AND (start row <= end row)
- Loop from start column to end column
- At result[start_row][i] assign counter variable.
- increment counter
- Increment start row
- Loop from start row to end row
- At result [i][end_column] assign counter variable
- Increment counter
- Decrement end row
- ... repeat for other two sides
- Loop from start column to end column
2. Solution
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 | function matrix(n) { const results = []; for (let i = 0; i < n; i++) { results.push([]); } let counter = 1; let startColumn = 0; let endColumn = n - 1; let startRow = 0; let endRow = n - 1; while (startColumn <= endColumn && startRow <= endRow) { // Top row for (let i = startColumn; i <= endColumn; i++) { results[startRow][i] = counter; counter++; } startRow++; // Right column for (let i = startRow; i <= endRow; i++) { results[i][endColumn] = counter; counter++; } endColumn--; // Bottom row for (let i = endColumn; i >= startColumn; i--) { results[endRow][i] = counter; counter++; } endRow--; // start column for (let i = endRow; i >= startRow; i--) { results[i][startColumn] = counter; counter++; } startColumn++; } return results; } | cs |