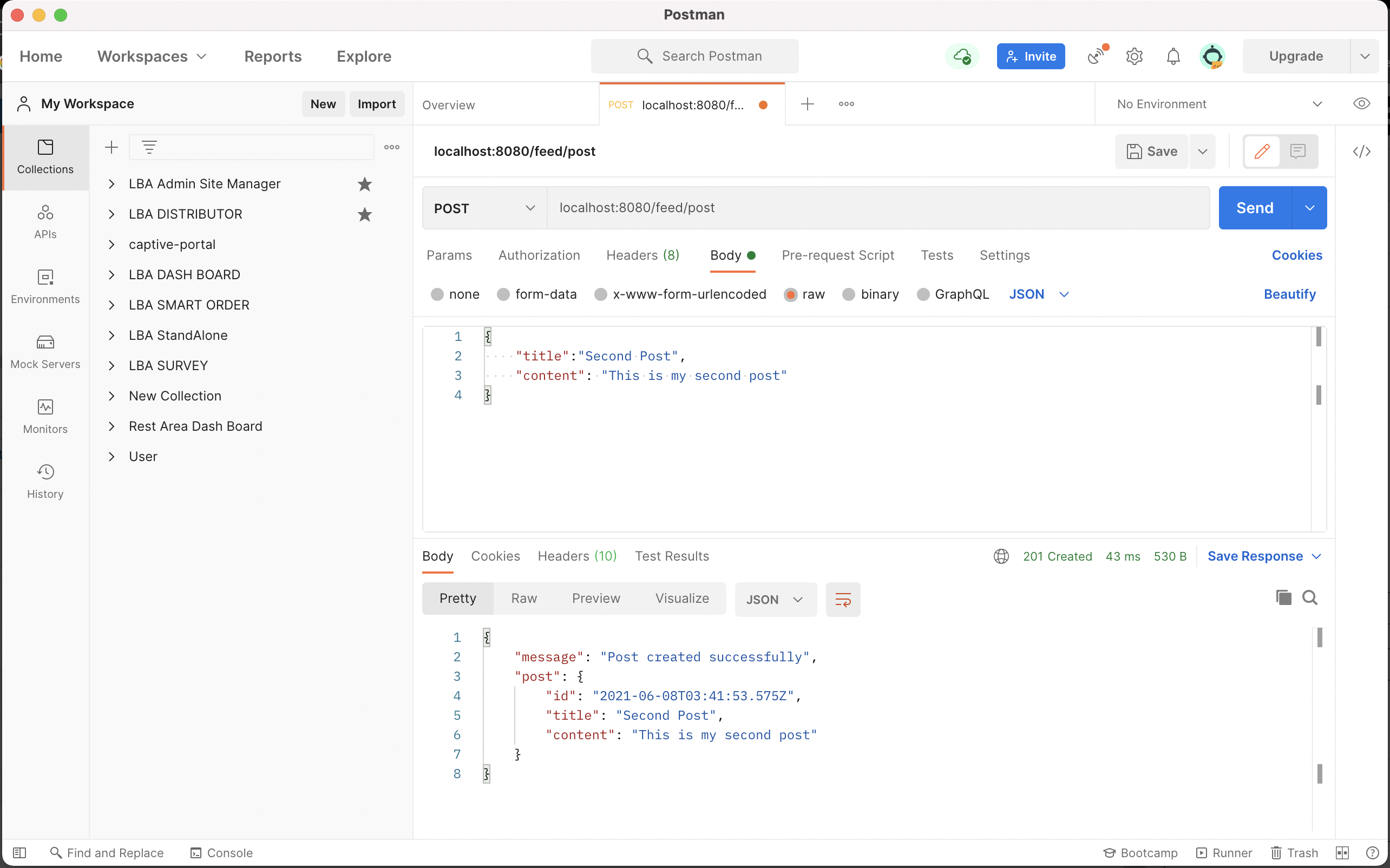
[Node js] Create Your Own Basic API
If you were working as a front end developer, you should image that how to make your own apis.
In this post, I will show your how to create your own api in your local server.
1. Create a Project Directory
1 2 3 4 5 | npm init npm install --save express body-parser npm install --save-dev node mon | cs |
in package.json script, add a start command
1 2 3 4 | "scripts": { "test": "echo \"Error: no test specified\" && exit 1", "start": "nodemon app.js" }, | cs |
2. Configure Middle Wares
First of all, you need you create app.js for our api. it will be our api's head office.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | const express = require('express'); const bodyParser = require('body-parser'); const feedRoutes = require('./routes/feed'); const { use } = require('./routes/feed'); const app = express(); app.use(bodyParser.json()); //application/json //will prevent to CORS Error app.use((req, res, next) => { res.setHeader('Access-Control-Allow-Origin', '*'); res.setHeader('Access-Control-Allow-Methods', 'GET, POST, PUT, PATCH, DELETE'); res.setHeader('Access-Control-Allow-Headers', 'Content-Type, Authorization'); next(); }) app.use('/feed', feedRoutes); //when clients use url/feed, this middle ware active the specific controller. app.listen(8080); | cs |
3. Create Route File.
Create Route foler and feed.js in your project file. This example api has two services, one is providing get posts list and the other is posting his/her own post
In your feed.js file should be like below
1 2 3 4 5 6 7 8 9 10 11 12 | const express = require('express'); const feedController = require('../controllers/feed'); const router = express.Router(); // Get /feed/posts router.get('/posts', feedController.getPosts); router.post('/post', feedController.createPost); module.exports = router; | cs |
4. Set Up a Controller
Controller has specific orders list when clients send a right request.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | exports.getPosts = (req, res, next) => { res.status(200).json({ posts: [{ title: "First Posts", content: "This is the first post!" }], }); }; exports.createPost = (req, res, next) => { const title = req.body.title; const content = req.body.content; console.log(title, content);l // Create post in db res.status(201).json({ message: "Post created successfully", post: { id: new Date().toISOString(), title: title, content: content }, }); }; | cs |
As you see that the getPost function, it will return the result which as status 200 and json type data. on createPost function. it will get a title and content data from the request and save the data into the local variable and return the result which has 201 status and json type data.
5. Let's Test!
Before we start our api, you must run our local node js server.
go to your directory and run "npm run start"
In my case, I used to use a Postman for test an api so in this time I also use it. if you had any other applications you used to, it would be okay.
Done! it's a simple api creation. you can do it as far as you want!