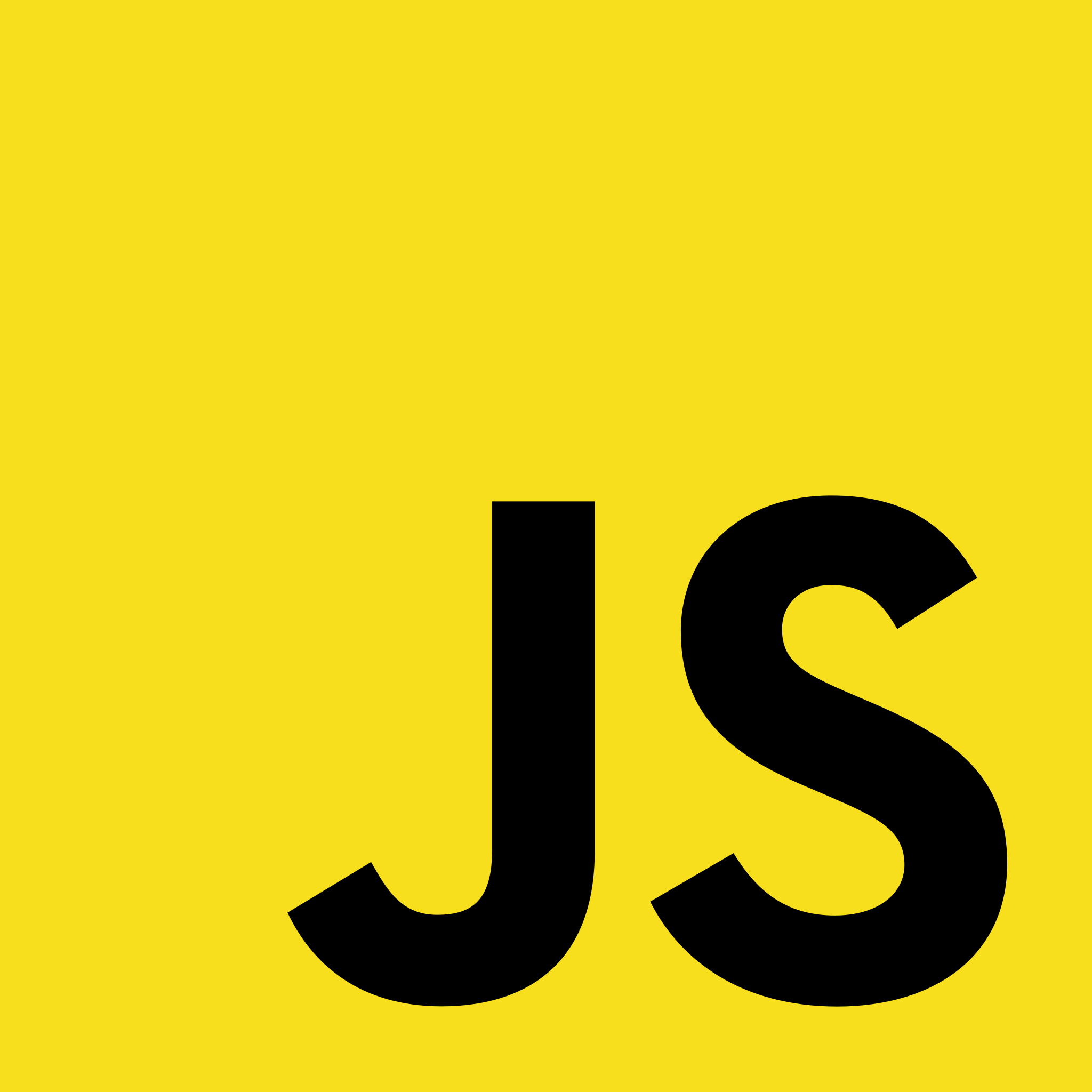
[Javascript Data Structure and Algorithms] Chapter2. Unique Parts
Chapter 2 JavaScript: Unique Parts
1. Javascript Scope
The scope is what defines access to JavaScript variables.
Global Declaration: Global Scope
In JavaScript, variables can be declared without using any operators.
However, this creates a global variable, and this is one of the worst practices in JavaScript. Avoid doing this at all costs.
Declaration with var: Functional Scope
In JavaScript, var is one keyword used to declare variables. These variable declarations "float" all the way to the top(variable hoisting).
This scope of the variable is the closest function scope. Let's see the next example
In Java, this syntax would have thrown an error because the insideIf variable is generally available only in that if statement block and not outside
the closest function scope means the scope is working under a function block instead of just the closest block
Declaration with let: Block Scope
Variable let is the closest block scope(meaning within the {} they were declared in)
In this example, nothing is logged to the console because the insideIf variable is available only inside the if statement block
2. Equality and Types
Variable Types
In JavaScript, there are seven primitive data types: boolean, number, string, undefined, object, function, and symbol.
3. Truthy/Falsey Check
Here are commonly used expressions that evaluate to false:
- false
- 0
- Empty string('' and"")
- NaN
- undefined
- null
4. === VS ==
JavaScript is a scripting language, and variables are not assigned a type during declaration. Instead, types are interpreted as the code run
Hence, === is used to check equality more strictly than ==, === checks for both the type and the value, while == checks only for the value.
5. Objects
Most strongly typed languages such as Java use is isEqual() to check whether two objects are the same. You may be tempted to simply use the == operator to check whether two objects are the same in JavsScript
However, this will not evaluate to be true.
Although these objects are equivalent, they are not equal. Namely, the variables have different addresses in memory.
This is why most JavaScript applications use utility libraries such as lodash or underscore, which have the isEqual(obj1, obj2) function to check two objects or values strictly.
Although the two functions perform the same operation, the functions have different addresses in memory, and therefore the equality operator returns false.
The primitive equality check operators, == and ===, can be used only for strings, and numbers.
6. Summery
JavsScript has a different variable declaration technique than most programming languages.
- var: function scope
- let/const block scope
- no declared: global scope
For type checking, typeof should be used to validate the expected type. Finally, for equality checks, use == to check the value, and === to check for the type as well as the value. However, use these only on non-object types such as numbers, strings, and booleans.