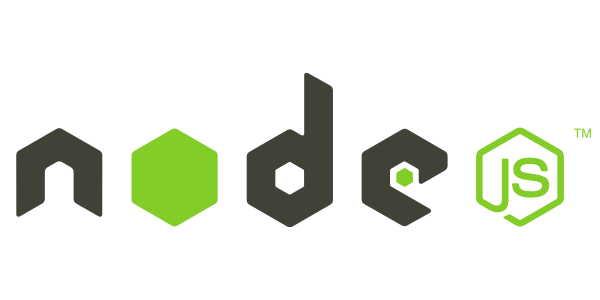
[Node.js] Testing Node.js Applications 2
Now Let's start to practical code test.
1. Test Preset
before we start to code test, you should set up some configurations.
- add test script into your package.json file"scripts": {"test":"mocha","start":"nodemon app.js"},
- create test directory and test.js file
2. Dummy Test
write down this code into your test.js file
it function is from mocha to set up your code test.
expect is chai function to test your code practically. There are several way to test your code in chai whether you can use expect function or should function. it's totally up to you.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | const expect = require('chai').expect; it('should add number correctly', function() { const num1 = 2; const num2 = 3; expect(num1 + num2).to.equal(5); }) it('should not give a result of 6', function() { const num1 = 3; const num2 = 3; expect(num1 + num2).not.to.equal(6); }) | cs |
then run your test code: npm test
3. Practical Test
Here is auth.js file In a middleware directory.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | const jwt = require('jsonwebtoken'); module.exports = (req, res, next) => { const authHeader = req.get('Authorization'); if (!authHeader) { const error = new Error('Not authenticated.'); error.statusCode = 401; throw error; } const token = authHeader.split(' ')[1]; let decodedToken; try { decodedToken = jwt.verify(token, 'somesupersecretsecret'); } catch (err) { err.statusCode = 500; throw err; } if (!decodedToken) { const error = new Error('Not authenticated.'); error.statusCode = 401; throw error; } req.userId = decodedToken.userId; next(); }; | cs |
Now we test the function in our new auth-middleware test file
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | const expect = require('chai').expect; const authMiddleware = require('../middleware/is-auth'); describe('Auth Middleware', function() { it('should throw an error if no authorization header is present', function() { const req = { get: function(headerName) { return null; } }; expect(authMiddleware.bind(this, req, {}, () => {})).to.throw('Not authenticated'); }); it('should throw an error if the authorization header is only one string', function() { const req = { get: function(headerName) { return 'xyz'; } }; expect(authMiddleware.bind(this, req, {}, () => {})).to.throw() } ) }) | cs |
- Describe function from Mocha distinguishes which functions are tested.
- There are two functions, one is check the header and auth-middleware throw a error message when the header has only string.
- Bind function is for preventing a error at Chai expect function because when you call the authMiddelware function directly, the expect function doesn't recognize what you send.
Now the npm test time!
This is the code testing in Node.js! Now you can easy to write your own test code yourself!