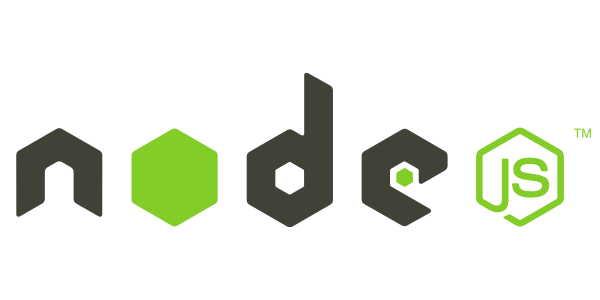
[Node Js] .Then Blocks to Async Await
When you call the async chains, How do you handle it? Do you still using it .then block? Yes it's a fundamental solution and async await block actually use .then blocks inside. However, if you use the async await, your colleagues are easy to understand your code!
1. Check Your Node Version
node -v
After Node 14.06, you can use async await syntax.
2. Your Parameters Will Be a Variable.
Await syntax cut the then blocks chains so you need to save your parameter into a variable.
If you don't need to save the return value such as end of the .then block chain. you don't need to save the value and just do it await function.
.then Syntax
1 2 3 4 5 6 7 8 9 10 11 12 13 | bcrypt .hash(password, 12) .then(hashedPw => { const user = new User({ email: email, password: hashedPw, name: name }); return user.save(); }) .then(result => { res.status(201).json({ message: 'User created!', userId: result._id }); }) | cs |
Async Await Syntax
1 2 3 4 5 6 7 8 | const hashedPw = await bcrypt.hash(password, 12); const user = new User({ email: email, password: hashedPw, name: name, }); const result = await user.save(); res.status(201).json({ message: "User created!", userId: result._id }); | cs |
3. .Catch Syntax Goes to Try Catch
.then Syntax
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | bcrypt .hash(password, 12) .then(hashedPw => { const user = new User({ email: email, password: hashedPw, name: name }); return user.save(); }) .then(result => { res.status(201).json({ message: 'User created!', userId: result._id }); }) .catch(err => { if (!err.statusCode) { err.statusCode = 500; } next(err); | cs |
Async Await Syntax
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | try { const hashedPw = await bcrypt.hash(password, 12); const user = new User({ email: email, password: hashedPw, name: name, }); const result = await user.save(); res.status(201).json({ message: "User created!", userId: result._id }); } catch (err) { if (!err.statusCode) { err.statusCode = 500; } next(err); } | cs |
Now do you have a confidence to change your then syntax to Async Await syntax? But don't forget how to use the then syntax! Behind Async Await syntaxes, then syntaxes are working on it for your readable codes.