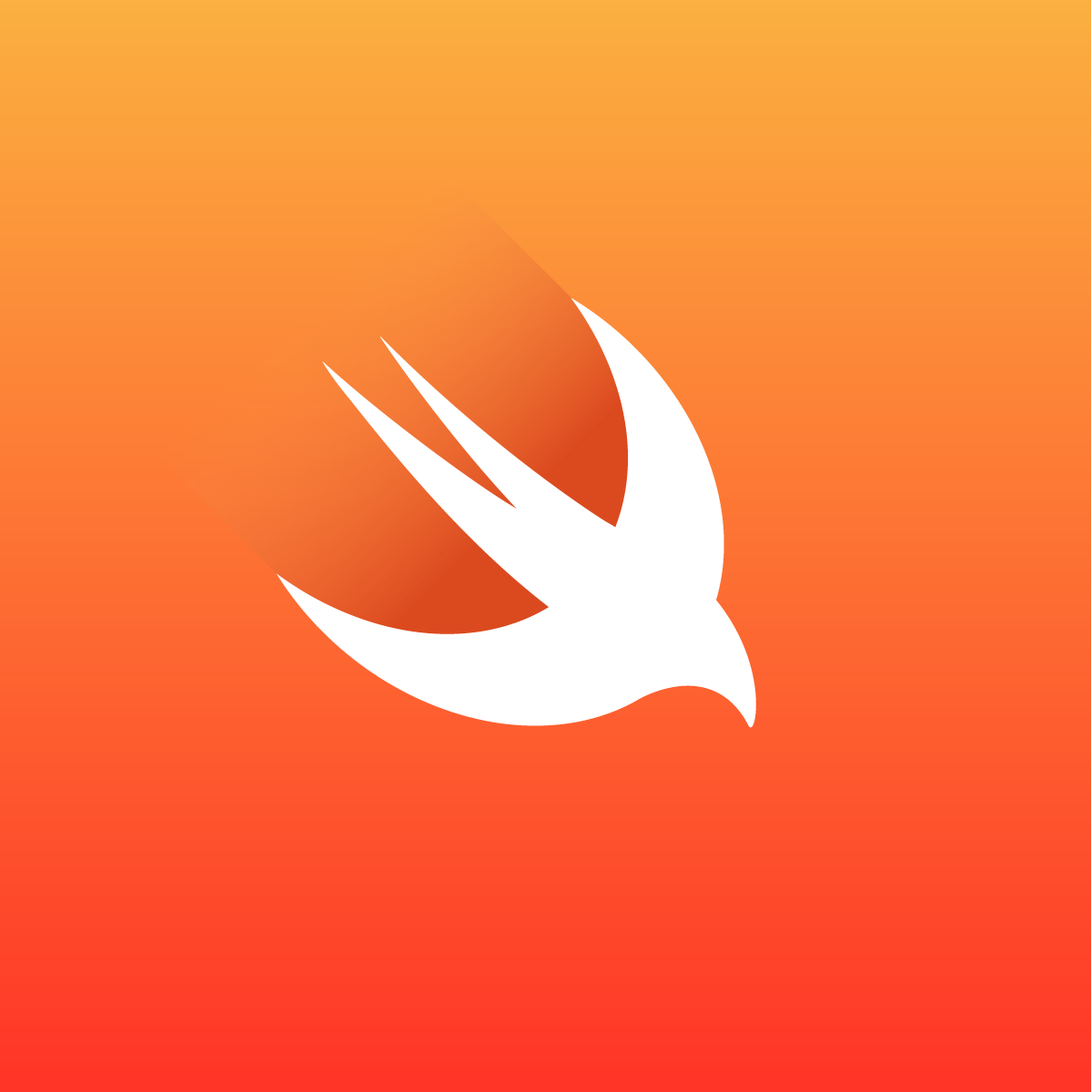
[Swift] Using Optionals
Optionals force to make variable holds specific types of values. For example, var test: String? means test can hold only String or nil value.
Optional concept prevent to developers mistake and concrete apps
1. Five Types of Optionals
- Force Wrapping
- Check for nil value
- Optional Binding
- Nil Coalescing Operator
- Optional Chaining
2. Details of Five Optionals
2-1) Force Wrapping
optional? (wrap) -> optional! (unwrap)
Wrapping means that optionals assign types of value into a variable. E.g. below example, var optionalValue: String? means optionalValue could only has String type data or nil.
var optionalValue: String?
optionalValue = nil
let text: String = optionalValue -> Error
let text: String = optionalValue! -> OK because String? and String is obviously different things so you need to unwrap the optionalValue to make it String
2-2) Check for nil value
1 2 3 | if optional != nil { optional! } | cs |
Check for nil value is using a if condition, check the optionalValue type and execute next code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | var optionalValue: String? optionalValue = nil if optionalValue != nil { let text: String = optionalValue! text = "test" let text2: String = text print(text2) } else { print("Always printed") } | cs |
but It's too wordy! so we need to move on next step.
2-3) Optional Binding
1 2 3 4 5 | if let safeOptional = optional { safeOptional } | cs |
1 2 3 4 5 | if safeOptional == optionalValue { let text: String = safeOptional } | cs |
2-4) Nil coalescing Operator
optional ?? defaultValue
1 2 3 4 5 6 7 | let optionalValue: String? optionalValue = nil let text:String = optionalValue ?? "I'm the default value" print(text) | cs |
Nil coalescing operator makes this whole wordy code to be simple.
you don't need to write a long code when you are using a nil coalescing operator
2-5) Optional Chaining
optional?.property
struct optional override whole the struct property, though you didn't assign a optional onto the property.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | struct MyOptional { var property = 123 func method() { print("I'm a struct method.") } } let myOptional: MyOptional? myOptional = MyOptional() print(myOptional!.property) | cs |
Even if the property variable is not an optional but struct optional proceeds it's properties. and the unwrapping struct optional cannot detect nil value.
so you need to fix it as below
print(myOptional?.property)