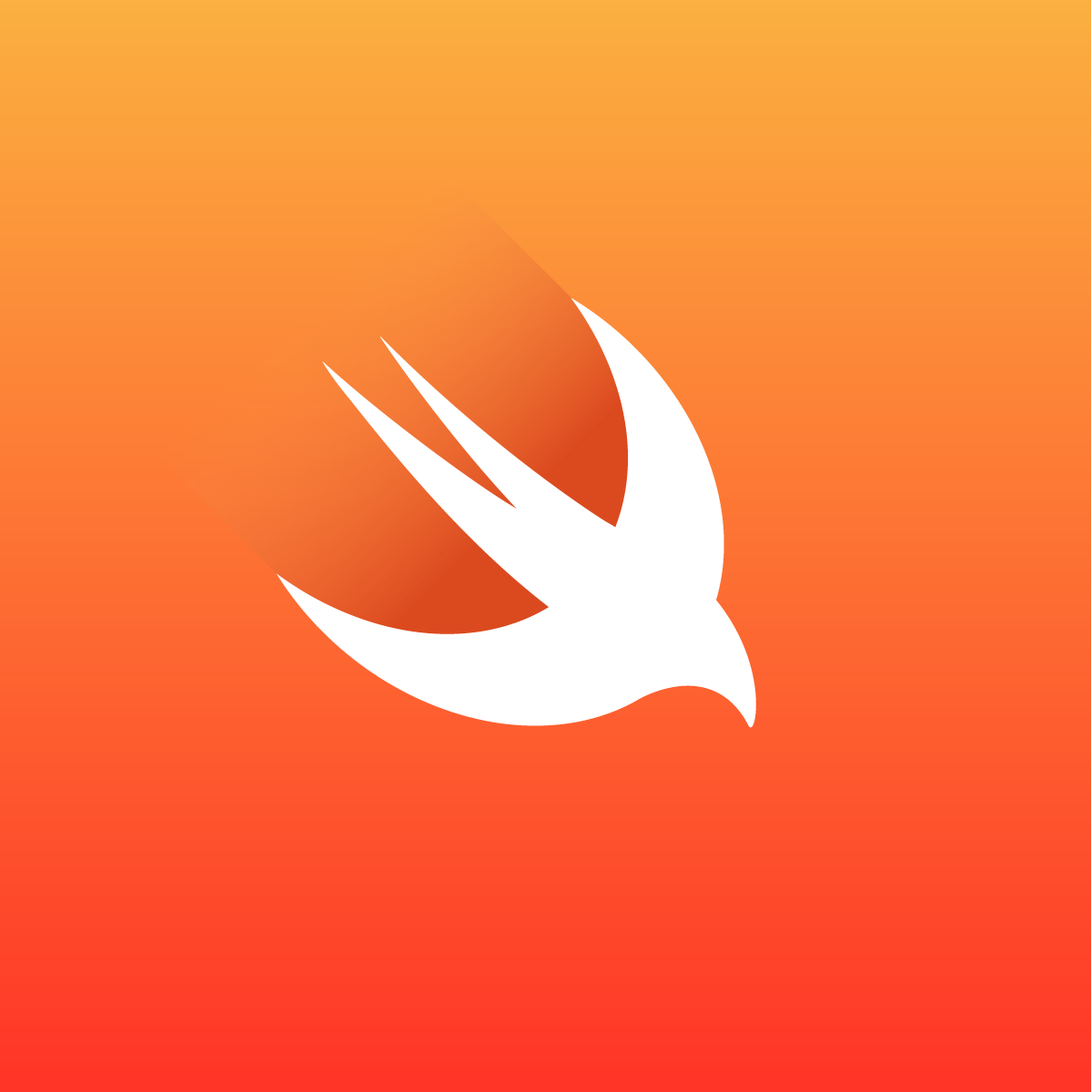
[Swift] Classes and Inheritance
1. Define the Class and Init
Define -> class MyClass { }
Init -> MyClass()
You can easy to replicated with classes!
Example on Enemy.swift
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | class Enemy { var health = 100 var attackStrength = 10 init(health: Int, attackStrength: Int) { self.health = health self.attackStrength = attackStrength } func takeDamage(amount: Int) { health = health - amount } func move() { print("Walk Forward.") } func attack() { print("Land a hit, dose \(attackStrength) damage.") } } | cs |
Main.swift
1 2 3 4 5 6 7 | let skeleton1 = Enemy(health: 100, attackStrength: 10) let skeleton2 = skeleton1 skeleton1.takeDamage(amount: 10) print(skeleton2.health) | cs |
2. Inheritance
Class childClass: SuperClass { }
SuperClass: Parents, they give whole properties and methods to subclasses
SubClass: Child of the Parents
Example, Create Dragon.swift
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | class Dragon: Enemy { var wingSpan = 2 func talk(speech: String) { print("Says: \(speech)") } override func move() { print("Fly forward.") } override func attack() { super.attack() print("Spits fire, dose 10 Damage") } } | cs |
Through override, you can re-writhe a methods as much as you want and super allows you can use whole properties of super class' properties.
3. How Swifts Use the Class Concepts?
UIButton class is a subclass of UIControl and it go back to back to back... you can reach out the NSObject. NSObject is the Super Class of whole the class. It inheritances whole the properties and methods which UIButton class has.