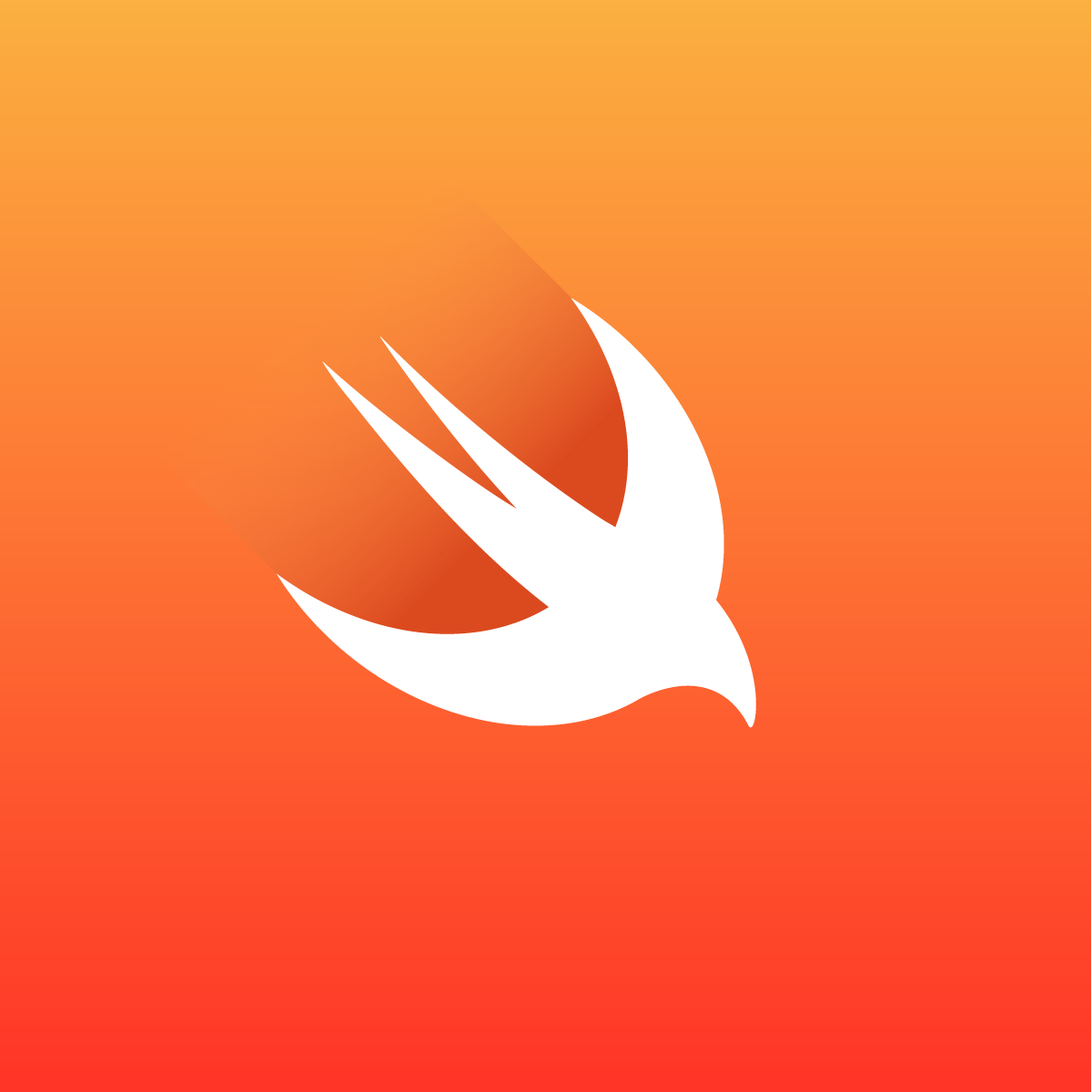
[Swift] Protocol, To Require Essentials
If you have essential properties and implements into selectively some classes instead of using an inheritance or struct, How could you solve this issue?
In swift, Protocol allows you to remove those problems. the protocol is requirements. When you add a protocol, you can use properties in the protocol whether objects's types are classes or structs.
1. This is a Problem.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 | protocol CanFly { func fly() } class Bird { var isFemale = true func fly() { print("The bird flaps its wings and lifts off into the sky.") } func layEgg() { if isFemale { print("The bird makes a new bird in a shell") } } } class Eagle: Bird { func soar() { print("The egale glide in the air using air currents.") } } class Penguin: Bird { func swim() { print("the penguin paddles through the water") } } struct FlyingMuseum { func flyDemo(flyObject: Bird) { flyObject.fly() } } class Airplane: Bird { } let myEagle = Eagle() let myPenguin = Penguin() let myPlane = Airplane() let museum = FlyingMuseum() museum.flyDemo(flyObject: myPlane) | cs |
Using an inheritance, you can print out myPlane's fly methods, Even if, the plane is not a bird.
2. Override Can be a Solution?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 | protocol CanFly { func fly() } class Bird { var isFemale = true func fly() { print("The bird flaps its wings and lifts off into the sky.") } func layEgg() { if isFemale { print("The bird makes a new bird in a shell") } } } class Eagle: Bird { func soar() { print("The egale glide in the air using air currents.") } } class Penguin: Bird { func swim() { print("the penguin paddles through the water") } } struct FlyingMuseum { func flyDemo(flyObject: Bird) { flyObject.fly() } } class Airplane: Bird { override func fly() { print("The airplane uses its engine to lift off into the air.") } } let myEagle = Eagle() let myPenguin = Penguin() let myPlane = Airplane() let museum = FlyingMuseum() museum.flyDemo(flyObject: myPlane) | cs |
If you add override function, you can fix the first problem. Let's dive in deep. Penguins are cannot fly, while the penguin class inherits fly methods from super class, defined Bird.
How do we solve both issues?
3. Protocols
Yes, our solution is a protocol. it requires properties which the protocol has.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 | protocol CanFly { func fly() } class Bird { var isFemale = true func layEgg() { if isFemale { print("The bird makes a new bird in a shell") } } } class Eagle: Bird, CanFly { func fly() { print("The eagle flaps its wings and lifts off into the sky.") } func soar() { print("The egale glide in the air using air currents.") } } class Penguin: Bird { func swim() { print("the penguin paddles through the water") } } struct FlyingMuseum { func flyDemo(flyObject: CanFly) { flyObject.fly() } } struct Airplane: CanFly { func fly() { print("The airplane uses its engine to lift off into the air.") } } let myEagle = Eagle() let myPenguin = Penguin() let myPlane = Airplane() let museum = FlyingMuseum() museum.flyDemo(flyObject: myPlane) | cs |
As far as you read that, when classes or structs get protocols, they should use properties came from the protocols.
You can use it on struct, multiple protocols, and combine with superclass!
4. Protocols Has Rules.
Of course, protocols has rules.
- when you use a protocol with super class, super class must be declared in front of protocols.
- Protocols are separated by colons. If you'd like to use several protocols, you have to distinguish each protocols by colon(,)